Surface() 함수를 인자중심으로 분석을 해봤습니다.
androidx.compose.material 패키지 안에 있는 함수로 다음처럼 설명이 되어 있습니다.
Material Design surface.
Material surface is the central metaphor in material design. Each surface exists at a given elevation, which influences how that piece of surface visually relates to other surfaces and how that surface casts shadows.
If you want to have a Surface that handles clicks, consider using another overload.
The Surface is responsible for:
Clipping: Surface clips its children to the shape specified by shape
Elevation: Surface draws a shadow to represent depth, where elevation represents the depth of this surface. If the passed shape is concave the shadow will not be drawn on Android versions less than 10.
Borders: If shape has a border, then it will also be drawn.
Background: Surface fills the shape specified by shape with the color. If color is Colors.surface, the ElevationOverlay from LocalElevationOverlay will be used to apply an overlay - by default this will only occur in dark theme. The color of the overlay depends on the elevation of this Surface, and the LocalAbsoluteElevation set by any parent surfaces. This ensures that a Surface never appears to have a lower elevation overlay than its ancestors, by summing the elevation of all previous Surfaces.
Content color: Surface uses contentColor to specify a preferred color for the content of this surface - this is used by the Text and Icon components as a default color.
Blocking touch propagation behind the surface.
If no contentColor is set, this surface will try and match its background color to a color defined in the theme Colors, and return the corresponding content color. For example, if the color of this surface is Colors.surface, contentColor will be set to Colors.onSurface. If color is not part of the theme palette, contentColor will keep the same value set above this Surface.
Params:
modifier - Modifier to be applied to the layout corresponding to the surface
shape - Defines the surface's shape as well its shadow. A shadow is only displayed if the elevation is greater than zero.
color - The background color. Use Color.Transparent to have no color.
contentColor - The preferred content color provided by this Surface to its children. Defaults to either the matching content color for color, or if color is not a color from the theme, this will keep the same value set above this Surface.
border - Optional border to draw on top of the surface
elevation - The size of the shadow below the surface. Note that It will not affect z index of the Surface. If you want to change the drawing order you can use Modifier.zIndex.
함수 정의
: Surface.kt 파일안에 다음처럼 정의되어 있습니다.
함수 내부 인자 테스트
: 기본 소스코드
Surface(
modifier = Modifier.padding(8.dp)
.fillMaxSize(),
border = BorderStroke(5.dp, Color.Red),
contentColor = Color.Yellow,
elevation = 1.dp,
shape = RectangleShape,
) {
Text(
text = "example",
modifier = Modifier.padding(2.dp)
.wrapContentSize()
.background(Color.Green)
)
}
▼ border
※ BorderStroke(1.dp , Color.Green)
※ BorderStroke(5.dp , Color.Red)
※ BorderStroke(10.dp , Color.Blue)
▼ shape
※ RectangleShape ( default value )
※ CircleShape
※ RoundCornerShape
※ CutCornerShape
▼ color
: 배경색 지정
※ Color.Gray
위 내용관련 코드는 아래와 같습니다.
Surface(
modifier = Modifier.padding(8.dp)
.fillMaxSize(),
border = BorderStroke(5.dp, Color.Red),
contentColor = Color.Black,
elevation = 0.dp,
shape = CircleShape,
color = Color.Gray,
) {
Text(
text = "example",
modifier = Modifier.padding(2.dp)
.wrapContentSize()
)
}
▼ contentColor
: 내부 자식 뷰에서 사용되는 content 색깔지정
자식 함수에서 색지정이 우선적으로 표시됩니다.
※ Color.Yellow
※ Color.Blue
※ Color.Red
※ Color.Green
▼ elevation
: 주변 요소와의 구별시 사용됩니다.
※ 0.dp (default)
※ 50.dp
※ 100.dp
▼ modifier
: 너무 많아서 중요한 항목만 정리 합니다.
※ padding
기본 소스코드
Surface(
border = BorderStroke(2.dp, Color.Red),
contentColor = Color.Black,
elevation = 0.dp,
modifier = Modifier
.fillMaxSize()
.wrapContentSize()
) {
Text(text = "example",)
}
>> no padding
.padding(10.dp) // 4방면에 10dp를 적용합니다.( Start/top/End/Bottom)
.padding(5.dp , 10.dp) // horizontal , vertical
.padding(10.dp , 5.dp , 2.dp, 1.dp) // Start , Top , End , Bottom
※ height / width
Surface(
border = BorderStroke(2.dp, Color.Red),
contentColor = Color.Black,
elevation = 0.dp,
modifier = Modifier
.width(150.dp)
.height(100.dp)
) {
Text(text = "example",)
}
.width() 미사용시 content 크기가 적용됩니다.
※ fillMaxWidth() , fillMaxHeight() ,fillMaxSize()
.fillMaxWidth() 적용
텍스트만 중간으로 이동할려면
Text(text = "example", textAlign = TextAlign.Center,)
.fillMaxHeight() 적용
==> 위화면에서 텍스트만 화면의 중간으로 이동하기
Text() 함수를 수정해야 합니다.
Text(text = "example", modifier = Modifier.wrapContentSize(Alignment.Center))
.fillMaxSize() : fillMaxWidth() 와 fillMaxHeight() 동시 적용시 사용합니다.
.wrapContentSize()
.wrapContentSize() && .fillMaxSize() 동시 적용
.wrapContentSize(align=Alignment.TopEnd, true)
.wrapContentSize(align=Alignment.CenterStart, true)
==> align 에 적용가능한 값
Alignment.TopStart,
.TopEnd ,
.CenterStart,
.CenterEnd
.BottomStart
.BottomEnd ,
.Size(100.dp)
.Size(100.dp , 40.dp) // width , height
.scale(scaleX , scaleY) >> Zoom in / out
.scale(1.5f , 0.8f)
.alpha(0.5f)
.alpha(0.1f)
.absoluteOffset(100.dp, 200.dp) && .fillMaxSize()
.clip(CircleShape)
<기타>
.fillMaxSize()
.wrapContentSize()
.drawWithCache {
val width =40.dp
val height = 40.dp
onDrawWithContent {
drawLine(Color.Red, Offset.Zero, Offset(width.toPx(), height.toPx()), 10f)
drawLine(Color.Blue, Offset.Zero, Offset(-width.toPx(), -height.toPx()), 4f)
}
}
복합 보더
Surface(
border = BorderStroke(2.dp, Color.Red),
contentColor = Color.Black,
elevation = 0.dp,
modifier = Modifier
.fillMaxSize()
.wrapContentSize()
.padding(8.dp) // margin
.border(2.dp, Color.Red) // outer border
.padding(8.dp) // space between the borders
.border(2.dp, Color.Green) // inner border
.padding(8.dp) // padding
.border(2.dp,Color.Blue, CircleShape)
) {
Text(text = "example")
}
그럼 오늘도 수고하세요.
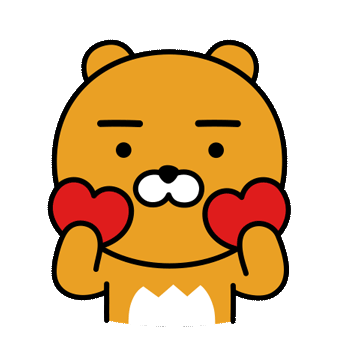
'Android_app' 카테고리의 다른 글
[JETPACK Compose] Column() 함수 내부 인자 테스트 (0) | 2022.11.21 |
---|---|
[JetPack Compose] Text() 함수 인자 분석 (0) | 2022.11.17 |
[Android Studio ] 스마트폰 화면 스크린 캡쳐 하기 (0) | 2022.11.15 |
[Android Studio] kotlin to Java 변환하기 (0) | 2022.11.01 |
[Android Studio] Build Error (0) | 2022.11.01 |